In this instructional guide, you will acquire the expertise to employ the PHP strpos() function effectively, enabling you to retrieve the position of the initial appearance of a specific substring within a given string.
Unlocking the Power of PHP’s strpos() Function
The PHP programming language offers a treasure trove of functions, each with its unique capabilities. One such gem in the PHP arsenal is the strpos() function, a versatile tool for string manipulation. In this comprehensive guide, we’ll dive deep into the world of strpos() and explore its syntax, parameters, and various use cases.
Syntax of strpos() Function
Let’s start by examining the syntax of the strpos() function:
strpos ( string $haystack , string $needle , int $offset = 0 ) : int|false
Parameters of strpos()
The strpos() function comes equipped with three essential parameters:
- $haystack: This is the string within which you want to search for a substring;
- $needle: The $needle is the string value you’re seeking within the haystack;
- $offset: An integer that represents the starting point for the search within the $haystack. It’s important to note that the $offset parameter is optional, and if omitted, it defaults to 0.
Now, let’s delve deeper into the nuances of the $offset parameter, as it plays a crucial role in how the strpos() function behaves.
Exploring the $offset Parameter
The $offset parameter in strpos() is like your starting point on a treasure map. Understanding how to wield it effectively can greatly enhance your string searching capabilities:
- Positive $offset: If you provide a positive integer as the $offset, the strpos() function will commence its search from that many characters into the $haystack, counting from the beginning of the string;
- Negative $offset: Conversely, when you use a negative integer as the $offset, strpos() will initiate the search by moving backward from the end of the $haystack, similar to retracing steps on a path.
Handling No Match Scenarios
In the adventurous journey of string searching, it’s crucial to be prepared for situations where your quest yields no results. In such cases, strpos() doesn’t return an error; instead, it gracefully provides feedback:
Return Value: If strpos() doesn’t locate the $needle within the $haystack, it returns false. This behavior allows you to handle such scenarios gracefully in your code. Also, discover the art of crafting dynamic dropdowns with PHP select option. Elevate your web development skills with our insightful guide!
Comprehensive Guide to PHP strpos() Function
PHP, a widely-used server-side scripting language, offers a variety of built-in functions, one of which is strpos(). This function is instrumental in string manipulation, specifically for locating the position of a substring within a string. Understanding how to effectively utilize strpos() can significantly enhance coding efficiency and capability in PHP.
1. Basic Usage of PHP strpos() for Substring Search
Purpose: The primary function of strpos() is to find the exact position of one string inside another.
Example Scenario: Consider a situation where you need to locate the first occurrence of the substring “do” within the phrase “To do or not to do”.
Implementation:
- You assign the string to a variable, say $str;
- Utilize strpos() by passing $str and the substring “do” as arguments;
- The function returns the index of the first appearance of “do”.
Code illustration:
$str = 'To do or not to do';
$position = strpos($str, 'do');
echo $position; // Outputs: 3
Output Explained: The output 3 signifies that the first occurrence of “do” starts at the 4th character of $str (index 3, as PHP indexes start from 0).
2. Advanced Use of strpos() with an Offset
Purpose: Beyond basic searches, strpos() can also start the search from a specified position in the string, known as the offset.
Example Scenario: To locate a subsequent occurrence of “do” in “To do or not to do”, starting from a certain point in the string.
Implementation:
- Again, assign the string to $str;
- This time, pass an additional argument to strpos() – the offset, which is the index from where the search should begin;
- The function then searches for “do”, starting from this offset.
Code illustration:
$str = 'To do or not to do';
$position = strpos($str, 'do', 4);
echo $position; // Outputs: 16
Output Explained: The output 16 indicates that starting from index 4, the next “do” is found at the 17th character of $str.
Mastering the PHP strpos() Function: Pitfalls and Solutions
The PHP strpos() function is a powerful tool for searching substrings within strings, but it comes with some gotchas that can lead to unexpected results. In this comprehensive guide, we’ll explore common pitfalls and provide you with valuable insights to make the most out of this function.
The Tricky Behavior of strpos()
Consider this example:
$str = 'To do or not to do';
$position = strpos($str, 'To');
if ($position) {
echo $position;
} else {
echo 'Not found';
}
Output:
Not found
At first glance, you might expect this code to output 0, as the substring ‘To’ starts at the beginning of the string. However, the strpos() function returns 0 to indicate a match at the start of the string, but PHP evaluates 0 as false. Hence, you see the ‘Not found’ message in the output.
Using the === Operator
To avoid falling into this trap, it’s essential to compare the result of the strpos() function correctly. Use the === or !== operator for precise comparisons. Here’s the improved code:
$str = 'To do or not to do';
$position = strpos($str, 'To');
if ($position !== false) {
echo $position; // 0
} else {
echo 'Not found';
}
Output:
0
By using !== false, you ensure that the result is explicitly compared to false, allowing you to correctly identify when a substring is found at the beginning of the string.
Exploring the PHP stripos() Function
When it comes to text manipulation and searching for substrings within strings in PHP, you have a powerful ally in the form of the stripos() function. This function is quite similar to its counterpart, strpos(), but with a significant twist – it conducts its search case-insensitively. In this comprehensive guide, we will delve deep into the workings of the stripos() function and provide you with valuable insights on how to make the most of it.
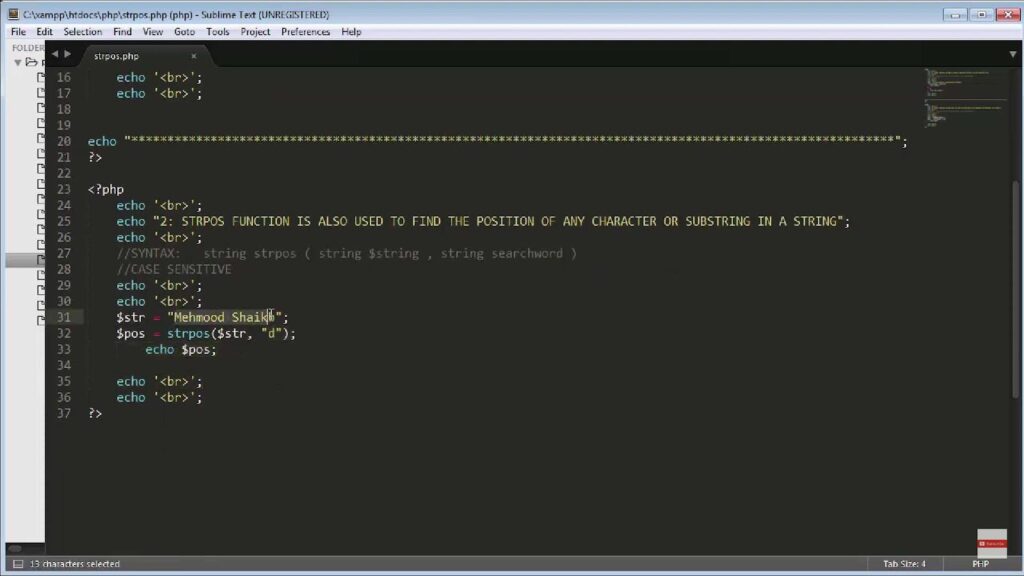
The Basic Syntax
The stripos() function takes two primary arguments: the haystack (the string in which you want to search) and the needle (the substring you are looking for). Here’s the basic syntax:
$position = stripos($haystack, $needle);
Now, let’s explore some key aspects and features of this function:
1. Case-Insensitive Search
As mentioned earlier, the standout feature of stripos() is its case-insensitive search capability. This means it will find a match regardless of whether the characters in the haystack and the needle are in uppercase or lowercase.
Example:
$str = 'PHP is cool';
$position = stripos($str, 'php');
var_dump($position); // Outputs: 0
In this example, even though ‘php’ is in lowercase within the haystack, stripos() successfully finds it, returning a position of 0.
2. Handling False Results
One crucial thing to note is that when stripos() fails to find a match, it returns false. This behavior differs from strpos(), which returns false only if the match is found at the beginning of the haystack.
Example:
$str = 'PHP is cool';
$position = stripos($str, 'python');
var_dump($position); // Outputs: false
In this case, ‘python’ is not present in the haystack, leading to a false result.
3. Additional Parameters
While the basic syntax covers the essentials, stripos() offers a couple of additional parameters that can enhance its functionality:
Offset: You can specify a starting point within the haystack from which the search should begin. This can be helpful if you want to skip a portion of the string.
$position = stripos($haystack, $needle, $offset);
Character Encoding: If you’re working with multibyte character encodings like UTF-8, you can specify the encoding to ensure accurate results.
$position = stripos($haystack, $needle, $offset, $encoding);
Conclusion
To conclude, this tutorial has equipped you with the knowledge and skills to proficiently utilize the PHP strpos() function for locating the index of the first instance of a desired substring within a provided string. With this newfound capability, you can enhance your PHP programming endeavors and tackle a wide range of string manipulation tasks with confidence.