In this comprehensive guide, you will delve into the art of harnessing the <select> element’s power to craft both dropdown menus and list boxes. Additionally, you will uncover the intricacies of extracting chosen values from the <select> element using PHP.
Unveiling the Power of the HTML <select> Element
The <select> element, a fundamental building block of web forms, empowers you to create interactive menus with ease. It’s your key to providing users with choices and enhancing their web experience. In this comprehensive guide, we will delve into the intricacies of the <select> element, its attributes, and how to make the most of it in your web development projects.
Defining a <select> Element in HTML
Creating a <select> element is straightforward. Let’s take a closer look at how to define one in HTML:
<label for="color">Background Color:</label>
<select name="color" id="color">
<option value="">--- Choose a color ---</option>
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
In this example, we’ve created a simple dropdown menu for selecting a background color. Users can choose between three options: Red, Green, and Blue. But there’s more to the <select> element than meets the eye.
Understanding Important Attributes
The <select> element boasts two essential attributes that play a crucial role in its functionality:
- id: The ‘id’ attribute establishes a connection between the <select> element and a corresponding <label> element. This connection enhances accessibility and improves user experience by associating the label with the input element. For example, the label with the ‘for’ attribute of ‘color’ is linked to the <select> element with the ‘id’ attribute of ‘color.’;
- name: The ‘name’ attribute is vital for form submissions. When a user makes a selection from the dropdown menu and submits the form, the ‘name’ attribute determines the name under which the selected value is sent to the server. It acts as the identifier for the selected option.
Crafting Options for the Menu
Now, let’s talk about the individual options within the <select> menu. Each <option> element nested inside the <select> element defines a choice available to the user. Here are some key points to keep in mind:
- Value Attribute: The ‘value’ attribute of each <option> element stores the data that will be submitted to the server when that particular option is selected. This data can be used for various purposes, such as processing user input or customizing the user experience;
- Default Value: If an <option> element lacks a ‘value’ attribute, it defaults to using the text contained within the <option> element as its value. This feature can be especially handy when the option text aligns with the data you want to collect.
Preselecting an Option
Sometimes, it’s desirable to preselect an option when the page first loads. To achieve this, you can use the ‘selected’ attribute within the <option> element. Let’s take a look at an example:
<label for="color">Background Color:</label>
<select name="color" id="color">
<option value="">--- Choose a color ---</option>
<option value="red">Red</option>
<option value="green" selected>Green</option>
<option value="blue">Blue</option>
</select>
In this case, the ‘Green’ option is selected by default when the page loads, thanks to the ‘selected’ attribute. This can be helpful in situations where you want to highlight a default choice or minimize user input.
Obtaining the Selected Value from a <select> Element
In this comprehensive guide, we will walk you through the process of creating a form that utilizes a <select> element in your web development project. This is a crucial skill for any web developer, as it enables you to interact with user choices and input effectively. Follow the steps below to get started and expand your knowledge:
Step 1: Setting Up Your Project Structure
Before diving into the coding, let’s create a well-structured project directory. This not only keeps your code organized but also makes it easier to maintain and collaborate with others.
Create the following folders and files within your project directory:
- css: This folder will contain your project’s stylesheet;
- style.css: Your project’s main stylesheet;
- inc: This directory will house various includes for your project;
- footer.php: The footer section of your HTML template;
- get.php: A PHP script to handle form data retrieval;
- header.php: The header section of your HTML template;
- post.php: A PHP script to handle form data submission;
- index.php: The main entry point of your web application.
Step 2: Setting Up the HTML Structure
Now that you’ve organized your project, it’s time to create the basic HTML structure. Let’s start by setting up the header in the header.php file. This file will be included at the beginning of all your web pages to ensure consistency in design and functionality.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="css/style.css">
<title>PHP Select Option</title>
</head>
<body class="center">
<main>
Here’s what each section does:
- <!DOCTYPE html>: Declares the document as an HTML5 document;
- <html lang=”en”>: Specifies the document’s language as English;
- <head>: Contains metadata about the document;
- <meta charset=”UTF-8″>: Defines the character encoding for the document;
- <meta name=”viewport” content=”width=device-width, initial-scale=1.0″>: Sets the viewport for responsive design;
- <link rel=”stylesheet” href=”css/style.css”>: Links the stylesheet for styling;
- <title>PHP Select Option</title>: Sets the title of the web page.
Step 3: Completing the HTML Structure
In the footer.php file, we complete the HTML structure:
</main>
</body>
</html>
This part concludes the main content of your web page. By including this footer in all your pages, you maintain a consistent layout and structure throughout your project.
Creating a Dynamic Color Selection Form:
In this section, we will guide you through creating a dynamic color selection form using HTML and PHP. This form will allow users to choose a background color, and upon submission, it will display their selection. Here’s a comprehensive breakdown of the process:
Step 1: Create the HTML Form (get.php):
To start, you need to create an HTML form that includes a dropdown menu (<select> element) for color selection and a submit button. Here’s the code for it:
<form action="<?php echo htmlspecialchars($_SERVER['PHP_SELF']) ?>" method="post">
<div>
<label for="color">Background Color:</label>
<select name="color" id="color">
<option value="">--- Choose a color ---</option>
<option value="red">Red</option>
<option value="green" selected>Green</option>
<option value="blue">Blue</option>
</select>
</div>
<div>
<button type="submit">Select</button>
</div>
</form>
Step 2: Submitting Data Using POST:
This form is set to use the POST method to submit data to the web server. This method ensures that sensitive information, such as the selected color, is not exposed in the URL.
Step 3: Processing the Submitted Data (post.php):
Next, you need to create a PHP script (post.php) to process the submitted data. Here’s the code for it:
<?php
$color = filter_input(INPUT_POST, 'color', FILTER_SANITIZE_STRING);
?>
<?php if ($color) : ?>
<p>You selected <span style="color:<?php echo $color ?>"><?php echo $color ?></span></p>
<p><a href="index.php">Back to the form</a></p>
<?php else : ?>
<p>You did not select any color</p>
<?php endif ?>
Enabling Multiple Selections in HTML Forms:
When you’re building interactive web forms, there may be situations where you want users to be able to select multiple options from a dropdown menu or list. This can be achieved by adding the “multiple” attribute to the <select> element in your HTML code. Let’s dive into how this feature works and how to implement it effectively.
How to Enable Multiple Selections:
To enable multiple selections in a <select> element, you simply add the “multiple” attribute. Here’s the basic syntax:
<select name="colors[]" id="colors" multiple>
<!-- Add your options here -->
</select>
Once you add the “multiple” attribute, users can select multiple options by holding down the Ctrl (or Command on macOS) key while clicking on the options they want to choose.
Handling Multiple Values:
When users select multiple options and submit the form, the form data for the <select> element will contain multiple values. To retrieve these values on the server-side, you need to use square brackets ([]) after the name attribute. In our example, the name is set to “colors[]”:
<select name="colors[]" id="colors" multiple>
<!-- Add your options here -->
</select>
This ensures that the selected values are sent as an array to the server.
An Example of Multiple Selections
Let’s explore a practical example of using a <select> element with multiple selections. In this example, we’ll create a simple web application step by step.
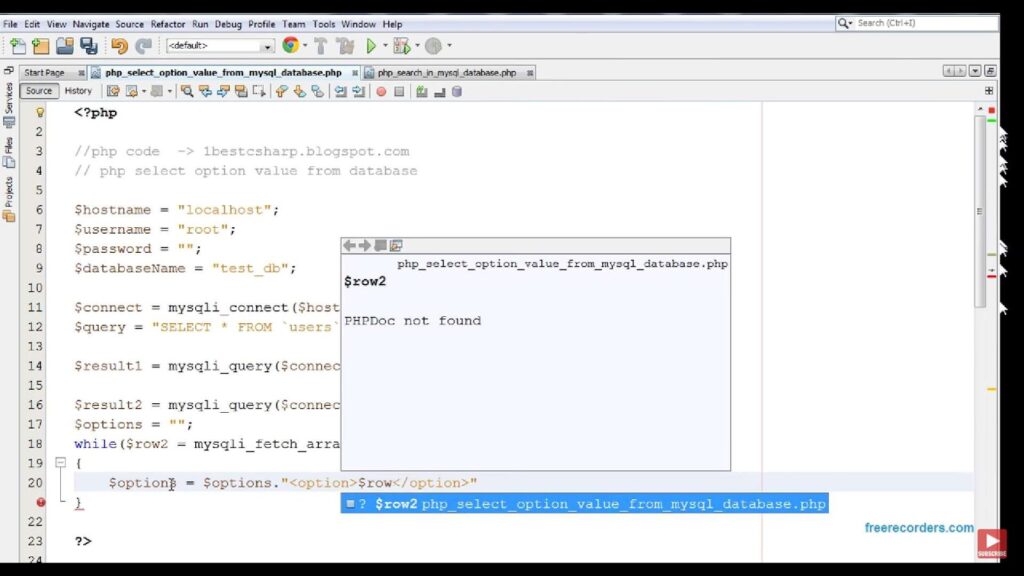
Step 1: Create the Folder Structure and Files:
Begin by organizing your project’s folder structure. This ensures clean and efficient code management. Here’s a suggested structure:
.
├── css
| └── style.css
├── inc
| ├── footer.php
| ├── get.php
| ├── header.php
| └── post.php
└── index.php
Step 2: Adding Code to header.php:
Now, let’s insert the necessary code into the header.php file. This file typically contains essential HTML elements that are shared across multiple pages of your website. Below is a sample code snippet for the header.php file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>PHP Listbox</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body class="center">
<main>
<!-- Your website content goes here -->
Step 3: Implementing Code in Footer
To enhance the functionality of your website, it’s crucial to include specific code in your footer.php file. This step ensures that your web pages are structured correctly and that essential elements are placed in the right locations. Here’s what you need to do:
</main>
</body>
</html>
This code should be added to your footer.php file, which is responsible for organizing the structure of your web pages. By including this snippet, you ensure that your web pages are correctly terminated and formatted.
Step 4: Integrating Header and Footer in Index
Now, let’s connect the dots and ensure a smooth flow between different parts of your website. By including the header.php and footer.php files in your index.php, you can create a cohesive and user-friendly experience for your visitors. Follow these steps:
<?php
require __DIR__ . '/inc/header.php';
$request_method = strtoupper($_SERVER['REQUEST_METHOD']);
if ($request_method === 'GET') {
require __DIR__ . '/inc/get.php';
} elseif ($request_method === 'POST') {
require __DIR__ . '/inc/post.php';
}
require __DIR__ . '/inc/footer.php';
In this section, we’ve incorporated PHP to dynamically load the appropriate content based on the HTTP request method. When a user visits your website, it will display a form from get.php if the request is GET. If a user submits the form, post.php will take care of processing the form submission. This dynamic approach makes your website more responsive and efficient.
Step 5: Creating a Dynamic Form
Now, let’s focus on creating an engaging and dynamic form that allows users to select multiple options. This form will be placed in the get.php file. Follow these guidelines:
<form action="<?php echo htmlspecialchars($_SERVER['PHP_SELF']) ?>" method="post">
<div>
<label for="colors">Background Color:</label>
<select name="colors[]" id="colors" multiple>
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
<option value="purple">Purple</option>
<option value="magenta">Magenta</option>
<option value="cyan">Cyan</option>
</select>
</div>
<div>
<button type="submit">Submit</button>
</div>
</form>
In this form, we’ve included a <select> element with the multiple attribute, allowing users to choose multiple background colors. The element’s name is wrapped in square brackets ([]), enabling PHP to handle the selected values as an array. This interactive form provides a user-friendly interface for your visitors.
Step 6: Handling Form Submissions
The final step involves processing the form submissions efficiently. This is done in the post.php file. Here’s how it’s done:
<?php
$selected_colors = filter_input(
INPUT_POST,
'colors',
FILTER_SANITIZE_STRING,
FILTER_REQUIRE_ARRAY
);
?>
<?php if ($selected_colors) : ?>
<p>You selected the following colors:</p>
<ul>
<?php foreach ($selected_colors as $color) : ?>
<li style="color:<?php echo $color ?>"><?php echo $color ?></li>
<?php endforeach ?>
</ul>
<?php else : ?>
<p>You did not select any color.</p>
<?php endif ?>
<a href="index.php">Back to the form</a>
In this step, the post.php file uses the filter_input() function to retrieve the selected colors as an array. If users have selected one or more colors, it will display them in an organized list with their respective colors. If no colors are selected, a message is displayed to inform the user.
Conclusion
To wrap up, this tutorial has equipped you with the knowledge to skillfully employ the <select> element, enabling you to fashion elegant dropdown lists and versatile list boxes. Furthermore, you’ve learned the essential techniques for retrieving selected values from the <select> element seamlessly through PHP. Armed with this newfound expertise, you are well-prepared to enhance your web development capabilities and create dynamic, user-friendly interfaces.