In this instructional guide, you will delve into the intricate workings of HTML forms and master the art of handling form data within the realm of PHP.
Comprehensive Guide to PHP Form Processing
When building web forms in PHP, the <form> tag is essential. This tag sets up the structure for user inputs and interactions on a webpage. Here’s a basic example:
<form action="form.php" method="post">
<!-- Form elements go here -->
</form>
This snippet is written in HTML/XML, which is the standard for web forms. The <form> element is characterized by two critical attributes:
- Action Attribute: This determines the destination URL for the form data upon submission. In the example, “form.php” is the script where the data is sent and processed;
- Method Attribute: This defines how data is sent to the server. The two most commonly used methods are:
- POST: Conceals data within the HTTP request; ideal for sensitive or large amounts of data;
- GET: Appends data to the URL; suitable for non-sensitive data and bookmarking pages.
It’s important to note that the method attribute is not case-sensitive. Both “post” and “POST” are valid. If omitted, the method defaults to GET.
Elements of a Form
Forms typically consist of various input elements, each serving a unique purpose:
- Text Fields: For single-line text inputs like names or email addresses;
- Password Fields: Similar to text fields but hide the input characters;
- Checkboxes: Allow multiple selections from several options;
- Radio Buttons: Enable a single selection from multiple choices;
- Dropdown Menus: Offer a list of options in a compact format;
- File Upload Controls: Facilitate the uploading of files.
Each input element within the form has essential attributes:
- Name: Crucial for identifying the element’s data in PHP;
- Type: Specifies the kind of input required (e.g., text, password, checkbox);
- Value: Represents the data entered or selected by the user.
The HTTP POST Method: Handling Form Data with PHP
When it comes to sending data from a web page to a server, the HTTP POST method is a crucial tool. This method allows you to securely transmit information by including it in the body of the HTTP request. In this comprehensive guide, we’ll delve into the ins and outs of the POST method and how you can effectively utilize it in your web development projects, particularly when working with PHP.
Understanding the POST Method
The POST method is one of the two primary methods in HTTP (the other being GET). It’s commonly used for submitting data to a server, such as when filling out forms on a web page. Unlike the GET method, which appends data to the URL, POST sends the data in the request body, making it a more secure and suitable choice for sensitive information.
Accessing Form Data in PHP
When a web form is submitted using the POST method, the data entered by the user is sent to the server. In PHP, you can access this data easily via the associative array $_POST. Each form field’s name becomes a key in this array, allowing you to retrieve the submitted values effortlessly.
For instance, if your form includes an input field with the name “email,” you can retrieve the email value in PHP using $_POST[’email’]. It’s important to note that if a particular input field is absent in the form, $_POST won’t contain an element with that specific key.
Checking the Existence of Form Data
Before working with form data, it’s essential to verify its existence. To do this, you can use the isset() function in PHP. This function helps you determine whether a specific key (representing a form field) exists in the $_POST array. Here’s how you can use it:
if (isset($_POST['email'])) {
// Process email
}
By employing this conditional statement, you ensure that you only process data that has been successfully submitted via the form.
Creating a Form with the POST Method
Let’s take a closer look at creating a basic HTML form that utilizes the POST method for data submission. Below is an example of a simple form:
<form action="form.php" method="post">
<div>
<label for="email">Email:</label>
<input type="email" id="email" name="email" />
</div>
<button type="submit">Submit</button>
</form>
In this example:
- The action attribute specifies the URL where the form data will be sent upon submission (in this case, “form.php”);
- The method attribute is set to “post,” indicating that the POST method will be used;
- The input field with the name “email” allows users to enter their email addresses.
Handling Submitted Data in PHP
Once the form is submitted, you can access the submitted data in the designated PHP file (in this case, “form.php”):
if (isset($_POST['email'])) {
var_dump($_POST['email']);
}
This code snippet checks if the “email” field exists in the $_POST array and, if so, displays its value using var_dump(). You can then process the data as needed, whether it’s storing it in a database, sending emails, or performing any other actions required for your web application.
Harnessing the Power of the HTTP GET Method in PHP
When it comes to processing data submitted through web forms in PHP, the HTTP GET method offers a unique and powerful approach. Unlike its counterpart, the POST method, GET method presents form data directly in the URL, allowing for easy retrieval and manipulation. In this comprehensive guide, we’ll delve into the intricacies of the HTTP GET method, explore its advantages, and equip you with the knowledge to effectively use it in your PHP projects.
Understanding the GET Method
The HTTP GET method, in essence, transforms your web form data into a part of the URL itself. This becomes evident when you submit a form using GET, and the URL is altered to include the form data as query parameters. Consider this example:
Original URL: http://localhost/form.php
Submitted URL: http://localhost/form.php?email=hello%40phptutorial.net
In this scenario, we’ve entered the email address “[email protected]” and submitted the form. Notice how the email value is appended to the URL, with special characters like “@” encoded as “%40.”
Benefits of Using the GET Method
- Ease of Debugging: Since the form data is visible in the URL, debugging becomes a breeze. You can instantly verify the data being sent and received;
- Bookmarkable URLs: GET parameters in the URL allow users to bookmark or share specific search results or data sets easily;
- Caching: GET requests are cacheable, which can significantly improve website performance by reducing server load.
Checking for GET Data in PHP
In PHP, you can conveniently check if your form data contains a particular element using the isset() function. Here’s a simple code snippet to illustrate this:
if(isset($_GET['email'])) {
// Process the email value
}
Handling Multiple Input Elements
When your form contains multiple input elements, the web browser appends them to the URL in a structured format. Each input’s name and its corresponding value are included as query parameters, separated by ampersands. For instance:
URL Structure: http://localhost/form.php?name1=value1&name2=value2&name3=value3
Implementing the GET Method in HTML Forms
To implement the GET method in your HTML form, specify it within the form element’s attributes. Here’s an example of an HTML form using the GET method for collecting email addresses:
<form action="form.php" method="get">
<div>
<label for="email">Email:</label>
<input type="email" id="email" name="email" />
</div>
<button type="submit">Submit</button>
</form>
Accessing GET Data in PHP
Once the form is submitted, you can access the GET data in your PHP script. It’s important to note that both $_POST and $_GET arrays are superglobal variables, meaning you can access them anywhere in your script. Here’s a basic example of how to access and display the email input:
if (isset($_GET['email'])) {
var_dump($_GET['email']);
}
Choosing Between HTTP GET and POST Methods
When it comes to sending data between a web form and a server, understanding when to use the HTTP GET or POST method is crucial. Let’s delve deeper into the differences and scenarios where each method is most appropriate.
GET Method: Retrieving Data
The HTTP GET method is the go-to choice when you’re building a form that solely aims to retrieve data from the server. Consider situations like search forms, where users seek information without causing changes to the server. Here’s why GET method shines:
- Fast and Efficient: GET requests are efficient for retrieving data because they are simple and can be cached by browsers and intermediaries;
- Bookmarked URLs: GET requests generate URLs that can be bookmarked or shared easily, making them ideal for search results or sharing specific content;
- No Side Effects: GET requests do not alter server-side data, ensuring a safe and predictable user experience.
POST Method: Triggering Server-Side Changes
On the flip side, the HTTP POST method is the weapon of choice when your form is meant to trigger server-side changes. Think of actions like submitting a newsletter subscription or making an online purchase. Here’s why POST method is the way to go:
- Secure Data Transmission: POST requests ensure that sensitive data, like passwords or payment information, is not exposed in URLs and is sent securely to the server;
- Large Data Submissions: When you need to transmit large amounts of data, such as file uploads or lengthy forms, POST can handle the task efficiently;
- Server-Side Processing: POST requests allow server-side scripts to process data, perform database updates, and maintain the integrity of server resources.
Example of a PHP Newsletter Subscription Form
Now, let’s explore a practical example to illustrate the concept further. Below is a PHP-based newsletter subscription form, showcasing the POST method in action:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="css/style.css">
<title>PHP Form Demo</title>
</head>
<body>
<main>
<form action="subscribe.php" method="post">
<div>
<label for="name">Name:</label>
<input type="text" name="name" required="required" placeholder="Enter your name" />
</div>
<div>
<label for="name">Email:</label>
<input type="email" name="email" required="required" placeholder="Enter your email" />
</div>
<button type="submit">Subscribe</button>
</form>
</main>
</body>
</html>
This form employs the POST method to send user-submitted data to the subscribe.php page for processing. It includes two input fields for capturing the user’s name and email address.
Client-Side Validation and Its Limitations
Before the form data is even sent to the server, modern web browsers perform client-side validation. This preliminary validation checks whether the required fields are filled out and if they adhere to the correct format. It’s a helpful user experience feature, but remember:
- HTML5 vs. JavaScript Validation: You can implement client-side validation using HTML5 attributes or JavaScript. HTML5 is simpler, while JavaScript provides more advanced validation capabilities;
- Not a Security Measure: Client-side validation is not a security measure. It primarily aids legitimate users in entering data correctly, but malicious users can bypass it.
Why Server-Side Validation Is Crucial
Server-side validation is an absolute necessity to ensure the integrity and security of your application. Client-side validation is a front-line defense, but server-side validation is your fortress. Here’s why:
- Security: Server-side validation prevents malicious users from injecting harmful data into your application;
- Data Integrity: It ensures that only valid and safe data reaches your server, maintaining data integrity;
- Consistency: Server-side validation guarantees that your application adheres to business rules and logic, even if client-side validation is bypassed.
Code Example: subscribe.php
Let’s take a look at the subscribe.php file, which processes the newsletter subscription form data:
<?php
//---------------------------------------------
// WARNING: this doesn't include sanitization
// and validation
//---------------------------------------------
if (isset($_POST['name'], $_POST['email'])) {
$name = $_POST['name'];
$email = $_POST['email'];
// Display a thank you message
echo "Thanks $name for your subscription.<br>";
echo "Please confirm it in your inbox of the email $email.";
} else {
// Notify the user to provide both name and email address
echo 'You need to provide your name and email address.';
}
Here’s how it works:
- It first checks if the name and email are present in the $_POST array using isset();
- If both are available, it displays a thank-you message along with email confirmation instructions;
- If not, it prompts the user to provide both their name and email address.
For instance, if a user submits the name as “John” and the email as “[email protected],” the subscribe.php page will respond with:
Thanks John for your subscription.
Please confirm it in your inbox of the email [email protected].
Enhancing Webpage Security: Safeguarding Against Cross-Site Scripting (XSS) Attacks
In the digital realm, web pages often need to display user-submitted data. However, this opens up a potential vulnerability, especially when a page naively displays form data without any preprocessing. Malicious actors can exploit this by injecting harmful scripts into these fields, compromising the integrity and security of the website. This is known as a Cross-Site Scripting (XSS) attack.
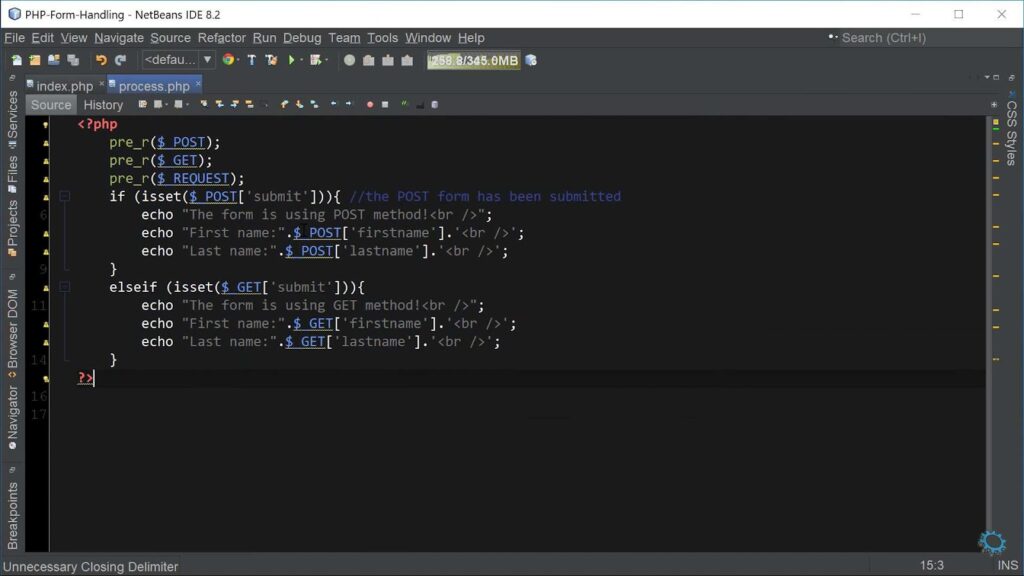
Real-World Scenario of XSS Vulnerability
Consider a scenario where a webpage, such as subscribe.php, is designed to capture user data like names and email addresses. If a user inputs a JavaScript snippet in a field, like the name input, it can lead to unexpected behavior. For instance:
- Input: <script>alert(‘Hello’);</script>;
- Result: An alert box pops up when the page loads.
The danger escalates if the script is designed to pull malicious code from an external source, directly affecting the user’s browser and potentially leading to severe security breaches.
Key Strategies for Mitigating XSS Risks
Escaping User Input: Before displaying any user input on a webpage, it’s crucial to “escape” this data. This process involves converting specific characters into their HTML-entities equivalents, ensuring they are displayed as text rather than executed as code.
Utilizing PHP’s htmlspecialchars() Function:
In PHP, htmlspecialchars() is a commonly used function for escaping user input.
Example usage:
if (isset($_POST['name'], $_POST['email'])) {
$name = htmlspecialchars($_POST['name']);
$email = htmlspecialchars($_POST['email']);
echo "Thanks $name for your subscription.<br>";
echo "Please confirm it in your inbox of the email $email.";
} else {
echo 'You need to provide your name and email address.';
}
Note: This code snippet only focuses on escaping and does not include data sanitization and validation. Read about unleashing your web development skills with our comprehensive guide on PHP contact forms. Make your first form now and enhance user interaction!
Conclusion
In conclusion, we’ve embarked on a journey through the intricate mechanics of HTML forms and unlocked the essential skills required to proficiently manage and manipulate form data in the dynamic world of PHP. With this newfound knowledge, you are now well-equipped to craft interactive and responsive web applications that cater to the needs of users far and wide.