PHP, a server-side scripting language, plays a vital role in building dynamic web pages. One key aspect that adds to its popularity among web developers is its ease of use and flexibility. A standout feature of PHP that deserves mention is the PHP OR operator. Let’s delve into its functionality and how it contributes to creating intricate logical expressions.
Exploring the Functions of the PHP OR Operator
In the realm of PHP, a server-side scripting language designed for web development, the logical OR operator is of prime importance. This operator takes two operands as input and returns a boolean response, i.e., true or false. The unique aspect of this operator is that it returns a true value if any of the operands hold true. Conversely, it will only produce a false value if both operands are false.
PHP recognizes the logical OR operator in two ways:
- Utilizing the ‘or’ keyword
expression1 or expression2;
- Using the || symbol
expression1 || expression2
The following table demonstrates the possible outcomes of employing the OR operator:
Expression 1 | Expression 2 | Expression 1 || Expression 2 |
---|---|---|
true | true | true |
true | false | true |
false | true | true |
false | false | false |
It’s essential to note that the use of ‘or’, ‘Or’, and ‘OR’ will yield the same outcomes since PHP is case-insensitive. This flexibility increases the user-friendliness of the language.
While ‘or’ and ‘||’ return the same result, they differ in their precedence levels. The ‘or’ operator possesses a higher precedence compared to ‘||’. It’s crucial to understand these differences to use these operators effectively.
Ways to Optimize the Use of the PHP OR Operator
Creating intricate logical expressions becomes less daunting with the PHP OR operator at your disposal. Here are some recommendations to enhance your utilization of this operator:
- Deepen your understanding of the logical expressions and how they interconnect with the PHP OR Operator;
- Setup the OR operator correctly within your PHP code, allowing for the resolution of multiple conditions simultaneously;
- Test the functionality of the PHP OR operator by creating both true and false conditions. This practice will give you a holistic understanding of the operator’s behavior in different scenarios;
- Familiarize yourself with common mistakes that can occur when using the PHP OR operator and learn efficient troubleshooting techniques.
Exploring Practical Examples of the PHP OR Operator
Consider a scenario where it’s required to clear the website cache if either of two conditions, represented by the flags $cacheExpired or $performPurge, is true. The logical OR operator becomes the perfect tool for this task, as shown below:
```php
<?php
$cacheExpired = true;
$performPurge = false;
$initiateCacheClear = $cacheExpired || $performPurge;
var_dump($initiateCacheClear);
```
This code will provide the following result:
bool(true)
This is because $cacheExpired is true, leading to a true result from the OR operator as well.
However, if the scenario changes and both $cacheExpired and $performPurge become false, the result will also be false. Here’s an example:
```php
<?php
$cacheExpired = false;
$performPurge = false;
$initiateCacheClear = $cacheExpired || $performPurge;
var_dump($initiateCacheClear);
```
This will yield the following outcome:
bool(false)
In real-world scenarios, the logical OR operator becomes incredibly useful in various conditional structures such as if, if-else, if-elseif, while, and do-while statements.
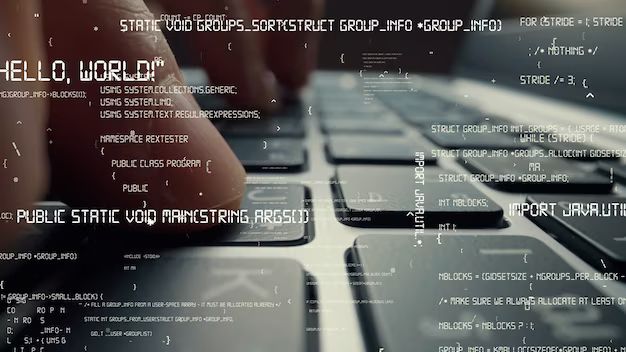
Unveiling the Concept of Short-Circuiting in PHP
One crucial aspect of the PHP OR operator is the idea of short-circuiting. Essentially, in the context of a logical OR operation, when the first operand proves to be true, the PHP interpreter bypasses the evaluation of the second operand entirely. This strategy, known as short-circuiting, enhances the speed and efficiency of the code, making it a valuable feature to comprehend and utilize.
A common pattern where the OR operator gets used is:
```
function_call() || die(message)
```
In this case, if function_call() returns true (indicating a successful function call), PHP will not execute the second operand — the die() function. Conversely, if the function call fails (returns false), PHP executes the die() function, outputting an error message.
For instance, consider the following code snippet:
```php
<?php
function connect_to_db()
{
return false;
}
connect_to_db() || die('Cannot connect to the database.');
```
In this example, the connect_to_db() function returns false, triggering PHP to execute the die() function, thus displaying the error message, “Cannot connect to the database.”
Demystifying Quirks in PHP OR Operator
When working with the PHP OR operator, developers occasionally encounter unexpected outcomes due to operator precedence. Understanding this peculiar trait of PHP might seem confusing initially but becomes straightforward once the underlying logic is discerned.
Consider the following PHP code:
<?php
$isTrue = false or true;
var_dump($isTrue);
In this scenario, one might assume that the output would be true since the operation (false or true) should return true. However, the outcome is false. This discrepancy arises due to operator precedence in PHP.
In the expression $isTrue = false or true;, PHP first evaluates $isTrue = false, and then processes the OR operator. This is because the assignment operator = holds a higher precedence than or.
Thus, the expression is technically equivalent to ($isTrue = false) or true;, leading to $isTrue being assigned false.
To rectify this and obtain the expected result, use parentheses to alter the order of evaluation:
<?php
$isTrue = (false or true);
var_dump($isTrue);
Alternatively, use the || operator, which has a higher precedence than =:
<?php
$isTrue = false || true;
var_dump($isTrue);
Both of these configurations will produce bool(true).
In Summation
In the end, understanding the nuances and intricacies of the PHP OR operator can significantly enhance your coding capabilities. This multifaceted operator has enormous potential, from simplifying complex logical expressions to optimizing code through short-circuiting or precedence quirks. Applying the knowledge gathered in this comprehensive guide can pave the way for success in mastering PHP and creating dynamic, robust web applications. If you’re aiming to enhance your programming expertise, it’s also advisable to delve into PHP File-Exists.