In this tutorial, we will cover the practical usage of the PHP array_map() function. This function is used to create a new array by applying a defined callback function to each element of the original array. It’s a valuable tool for data transformation and manipulation in PHP programming, making it a useful addition to your skillset.
While discussing the array_map function in PHP, it’s essential to understand its usage and potential benefits, which can also be applied when working with PHP substrings in various scenarios.
PHP’s array_map() Function for Array Transformation
In this tutorial, we will explore the functionality of the PHP array_map() function, which is particularly useful for transforming data within arrays.
Suppose you have an array containing the lengths of squares, like this:
<?php
$lengths = [10, 20, 30];
// calculate areas
$areas = [];
foreach ($lengths as $length) {
$areas[] = $length * $length;
}
print_r($areas);
The output would be:
Array
(
[0] => 100
[1] => 400
[2] => 900
)
This code iterates through the elements of the $lengths array, computes the area of each square, and stores the results in the $areas array.
Alternatively, you can achieve the same result using the array_map() function:
<?php
$lengths = [10, 20, 30];
// calculate areas
$areas = array_map(function ($length) {
return $length * $length;
}, $lengths);
print_r($areas);
In this example, array_map() applies an anonymous function to each element of the $lengths array, creating a new array containing the results of the anonymous function.
Starting from PHP 7.4, you can use an arrow function instead of an anonymous function:
<?php
$lengths = [10, 20, 30];
// calculate areas
$areas = array_map(
fn ($length) => $length * $length,
$lengths
);
print_r($areas);
This code demonstrates the more concise syntax introduced in PHP 7.4 for achieving the same array transformation with array_map().
The Syntax and Usage of PHP’s array_map() Function
In PHP, the array_map() function serves as a powerful tool for manipulating arrays. Let’s delve into its syntax and explore how it can be used effectively.
The array_map() function is structured as follows:
array_map ( callable|null $callback , array $array , array …$arrays ) : array
This function encompasses the following parameters:
- $callback: This parameter expects a callable function that will be applied to each element in the provided arrays;
- $array: An array of elements to which the callback function will be applied;
- $arrays (optional): A variable list of additional array arguments to which the callback function will also be applied.
The array_map() function operates by returning a new array. This array contains elements that are the result of applying the specified callback function to each corresponding element in the input arrays.
This tutorial primarily focuses on the following variation of the array_map() function:
array_map ( callable $callback , array $array ) : array
This version of the function takes a single array and applies the designated callback function to its elements, resulting in a new array containing the outcomes of this operation. We will delve deeper into the practical use of this particular form of array_map() to help you understand how it can streamline your array manipulation tasks.
Practical Examples of the PHP array_map() Function
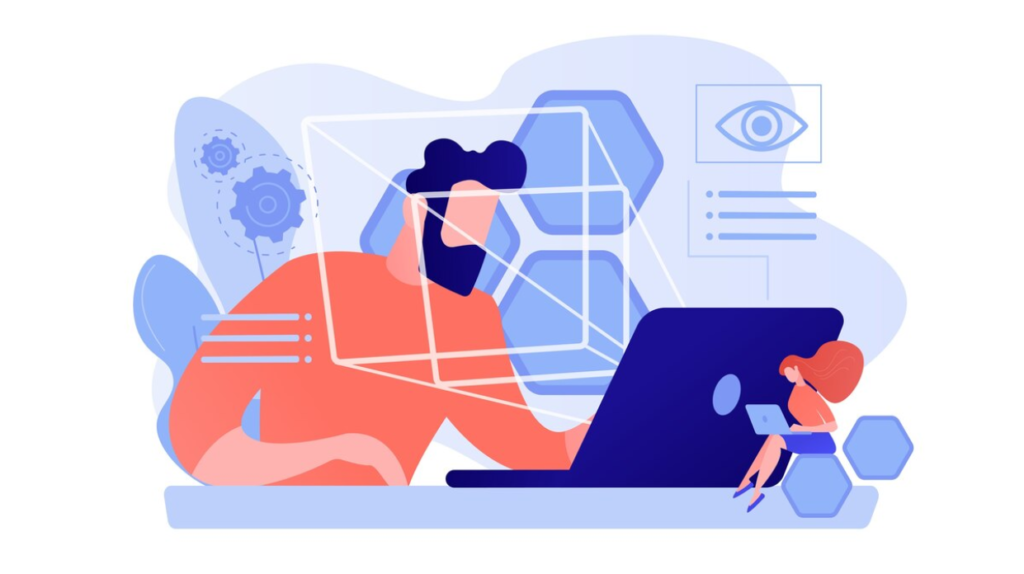
Let’s explore additional examples of how to utilize the array_map() function in PHP.
Employing PHP’s array_map() Function with an Array of Objects
In this example, we’ll introduce a class with three properties: $id, $username, and $email, and a list of User objects.
<?php
class User
{
public $id;
public $username;
public $email;
public function __construct(int $id, string $username, string $email)
{
$this->id = $id;
$this->username = $username;
$this->email = $email;
}
}
$users = [
new User(1, 'joe', '[email protected]'),
new User(2, 'john', '[email protected]'),
new User(3, 'jane', '[email protected]'),
];
The following demonstrates how to utilize the array_map() function to extract a list of usernames from the $users array:
<?php
class User
{
public $id;
public $username;
public $email;
public function __construct(int $id, string $username, string $email)
{
$this->id = $id;
$this->username = $username;
$this->email = $email;
}
}
$users = [
new User(1, 'joe', '[email protected]'),
new User(2, 'john', '[email protected]'),
new User(3, 'jane', '[email protected]'),
];
$usernames = array_map(
fn ($user) => $user->username,
$users
);
print_r($usernames);
Result:
Array
(
[0] => joe
[1] => john
[2] => jane
)
Utilizing a Static Method as a Callback
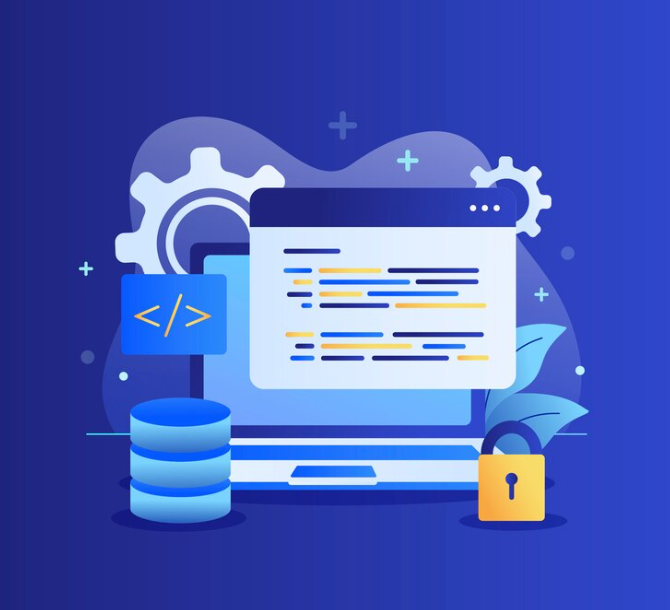
You can utilize a public method of a class as the callback function argument for the array_map() function. For instance:
<?php
class Square
{
public static function area($length)
{
return $length * $length;
}
}
$lengths = [10, 20, 30];
$areas = array_map('Square::area', $lengths);
print_r($areas);
How It Operates:
- To begin, define the Square class, incorporating the public static method called area();
- Next, establish an array to store the lengths of three squares;
- Proceed to compute the areas of these squares based on the lengths stored in the $lengths array, making use of the static method Square::area().
It’s worth noting that when passing a public static method as a callback to the array_map() function, you should adhere to the following syntax:
'className::staticMethodName'
Additionally, it’s essential that the public static method accepts the array element as an argument.
Conclusion
The PHP array_map() function proves to be an indispensable tool for any programmer. It allows the creation of new arrays by applying a specified callback function to every element of an existing array. This guide has dived deep into its syntax, provided examples for better understanding, and highlighted some advanced uses and best practices. Whether you’re just starting out or an experienced PHP developer, the flexibility and efficiency of the array_map() function is sure to become an essential part of your coding toolkit.