In this instructional guide, you will acquire the knowledge of integrating code from an external file by harnessing the power of the PHP include construct.
Exploiting the Power of the PHP Include Construct
PHP, a widely used scripting language, houses a multitude of constructs to simplify and enhance coding routines. One such potent construct is ‘include’.
The ‘include’ construct is best understood as a method to import code from a different file into the current file. This can save time and effort by allowing for code reuse and modularity.
Here’s a closer look at how it works and how to use it.
include 'path_to_file';
In the syntax above, after the ‘include’ keyword, the path to the desired file is placed. For instance, if you wish to import the code from a ‘functions.php’ file into another called ‘index.php’, the following ‘include’ statement will do the trick:
<?php
// Inside index.php file
include 'functions.php';
When PHP fails to locate the ‘functions.php’ file in the specified directory, it triggers a warning. The warning may look similar to this:
Warning: include(functions.php): failed to open stream: No such file or directory in … on line 4
Warning: include(): Failed opening ‘functions.php’ for inclusion (include_path=’\xampp\php\PEAR’) in … on line 4
While searching for the ‘functions.php’ file to load, PHP commences the search journey from the directory denoted by ‘include_path’. In this instance, it’s ‘\xampp\php\PEAR’. If PHP successfully locates the ‘functions.php’ file here, it proceeds to load the code from the file.
To streamline your PHP coding, observe the following tips:
- Make sure to correctly specify the path of the file to be included. If the path is incorrect or the file is missing, PHP will throw an error;
- Keep your code as modular as possible. This means separating functions, classes, or blocks of code that perform a specific task into different files. This makes your code more readable, reusable, and maintainable;
- Be mindful of PHP’s order of precedence when including files. PHP will first check the specified path, then the directory of the PHP script making the include call, and finally the server’s directory path;
- Take advantage of PHP’s error handling capabilities to handle possible inclusion errors. This could involve logging errors to a file for later review or displaying a user-friendly error message on the front-end.
A Deeper Dive into PHP’s ‘Include’ Construct
The PHP include construct, an invaluable tool for programmers, grants the ability to integrate code from one file into another seamlessly. This allows for reusable code snippets that can improve workflow, reduce redundancy, and enhance code management.
The ‘include’ construct uses a straightforward syntax:
include 'file_path';
In this structure, ‘file_path’ represents the location of the file from which the code will be drawn. As an illustration, let’s take a scenario where the code from ‘functions.php’ is to be imported into ‘index.php’. The correct ‘include’ statement would then be:
<?php
// Inside index.php
include 'functions.php';
In the event that PHP cannot find the ‘functions.php’ file at the specified location, it responds with a warning. Here is an example of what such a warning may look like:
Warning: include(functions.php): failed to open stream: No such file or directory in …
Warning: include(): Failed opening ‘functions.php’ for inclusion (include_path=’\xampp\php\PEAR’) …
The ‘include_path’ signals the directory that PHP first checks when searching for the ‘functions.php’ file. In our case, it is ‘\xampp\php\PEAR’. If PHP locates the ‘functions.php’ file here, it proceeds to load its code.
If it fails to find the file in the aforementioned directory, PHP next searches in the directory of the script calling the ‘include’ construct and the server’s current working directory. If found, PHP successfully loads the code from the ‘functions.php’ file.
An important aspect to note is that PHP doesn’t merely load the code from the ‘functions.php’, it also executes it. Let’s consider this segment of code in ‘functions.php’:
<?php
// Inside functions.php
function display_copyright() {
return 'Copyright © ' . date('Y') . ' by phpMaster.net. All rights reserved!';
}
echo display_copyright();
Upon including ‘functions.php’ into ‘index.php’ and loading ‘index.php’, the following output will be displayed:
Copyright © 2021 by phpMaster.net. All rights reserved!
This shows that not only did PHP load the code from ‘functions.php’, but it also executed it.
Here are some tips to master the use of ‘include’ in PHP:
- Always ensure the file path is correct to avoid inclusion errors;
- Code modularity is key. Place different functions or classes in separate files for improved reusability and maintainability;
- Understand PHP’s order of precedence in looking for files to include: the specified directory, the directory of the calling script, and the server’s path;
- Leveraging PHP’s error handling capabilities can help deal with possible inclusion errors in a more robust way.
The ‘include’ construct, when used properly, can greatly enhance the readability, reusability, and structure of your PHP code. Embrace it and let your coding efficiency soar! Read about the wonders of PHP’s strpos function and its string searching magic. Discover strpos in action!
Putting PHP’s ‘Include’ to Work: A Practical Example
Let’s now dive into an application-oriented overview of the PHP ‘include’ construct. A common use-case for ‘include’ is in managing recurring site design elements such as headers and footers across a multi-page website.
Consider a website with numerous pages, all sharing a standard header and footer. By placing the header and footer code into separate PHP files – ‘header.php’ and ‘footer.php’, for instance – you eliminate the need for repetition. Instead, you simply incorporate these files into each webpage with PHP’s ‘include’ construct.
This methodology not only promotes DRY (Don’t Repeat Yourself) principles, but it also greatly facilitates site-wide updates to the header and footer. Changes made to ‘header.php’ or ‘footer.php’ automatically propagate to every page that includes them.
Usually, it’s beneficial to place such recurring elements or templates in a separate directory for organized access. By tradition, this directory is often called ‘inc’:
.
├── index.php
├── functions.php
├── inc
│ ├── footer.php
│ └── header.php
└── public
├── css
│ └── style.css
└── js
└── app.js
In the directory structure above, ‘header.php’ and ‘footer.php’ are stored in the ‘inc’ directory, while the ‘public’ directory houses additional resources like CSS and JavaScript files.
The ‘header.php’ file embodies the page’s header code and may link to a stylesheet in the ‘public/css’ directory, as shown below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="public/css/style.css">
<title>PHP 'Include' Demonstration</title>
</head>
<body>
With the ‘include’ construct, the code from ‘header.php’ and ‘footer.php’ can be readily incorporated into any webpage. To drive this point home, let’s include the header and footer files into our ‘index.php’ file:
<?php
// Inside index.php
include 'inc/header.php';
// Page's main content here
include 'inc/footer.php';
By using the PHP include statement, the ‘index.php’ file now includes the header and footer code without any redundancy. This approach is a practical testament to the power and convenience brought to your PHP coding through the ‘include’ construct.
Remember, the ‘include’ construct is not only limited to headers and footers. It can be instrumental in including any reusable code pieces—like navigation bars, sidebars, or modals—across your PHP project.
The key is to streamline your PHP coding routines, enhance the organization, and uphold the DRY principles. Incorporate the ‘include’ construct into your PHP coding toolkit, and it will open doors to cleaner, smarter, and more efficient coding practices.
Illustrating the Power of PHP’s ‘Include’: A Step-by-Step Example
The use of ‘include’ in PHP can be truly grasped by exploring a hands-on example. Assuming a website layout with a uniform header and footer across all pages, the ‘include’ construct can prevent any redundant coding.
By placing the header and footer code in individual files (let’s call them ‘header.php’ and ‘footer.php’), they can be included in each page of the site without having to rewrite the code. This approach is a perfect testament to the practicality of the ‘include’ construct in PHP.
To maintain an organized project structure, it’s common to store such reusable template files like ‘header.php’ and ‘footer.php’ in a dedicated folder. A conventionally accepted name for this directory is ‘inc’:
.
├── index.php
├── functions.php
├── inc
│ ├── footer.php
│ └── header.php
└── public
├── css
│ └── style.css
└── js
└── app.js
In this arrangement, ‘header.php’ contains the code for the webpage’s header section, including a link to a stylesheet (‘style.css’) located in the ‘public/css’ directory:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="public/css/style.css">
<title>PHP 'Include' Usage Illustrated</title>
</head>
<body>
Now, let’s assume ‘footer.php’ holds the code representing the page’s footer:
<script src="public/js/app.js"></script>
</body>
</html>
With the ‘header.php’ and ‘footer.php’ files prepared, they can be effortlessly included in the main ‘index.php’ file:
<?php include 'inc/header.php'; ?>
<h1>Understanding PHP 'Include'</h1>
<p>This example demonstrates how the PHP 'include' construct operates.</p>
<?php include 'inc/footer.php'; ?>
Running ‘index.php’ and inspecting the output’s source code, you will observe the seamless integration of the ‘header.php’ and ‘footer.php’ files:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="public/css/style.css">
<title>PHP 'Include' Usage Illustrated</title>
</head>
<body>
<h1>Understanding PHP 'Include'</h1>
<p>This example demonstrates how the PHP 'include' construct operates.</p>
<script src="public/js/app.js"></script>
</body>
</html>
This simple but practical example showcases the ‘include’ construct’s potency in PHP and its utility in crafting consistent, reusable, and maintainable code. Remember, this is just one of many potential use-cases for ‘include’. It can be used in multiple diverse scenarios to improve your coding efficiency and effectiveness.
Understanding Variable Scopes with PHP’s ‘Include’
An essential aspect of using PHP’s ‘include’ construct is understanding how it interacts with variable scopes. When a file is included, all variables defined within that file inherit the variable scope of the line where the inclusion occurs.
Example: Inclusion Outside a Function
Consider this scenario: a file named ‘content.php’ defines two variables, $title and $bodyText.
<?php
// content.php
$title = 'PHP Include Explained';
$bodyText = 'This article illustrates the operation of the PHP include construct.';
If ‘content.php’ is included in another file, say ‘article.php’, both $title and $bodyText become global variables within ‘article.php’. Therefore, these variables can be used within ‘article.php’ as shown in the following snippet:
<?php include 'inc/header.php'; ?>
<?php include_once 'content.php'; ?>
<h1><?php echo $title; ?></h1>
<p><?php echo $bodyText; ?></p>
<?php include 'inc/footer.php'; ?>
In the above code, ‘article.php’ includes both ‘header.php’ and ‘footer.php’ for the page’s layout. Additionally, it includes ‘content.php’, allowing it to access and display the $title and $bodyText variables.
Remember, this is just one example of how ‘include’ interacts with variable scopes. It’s important to consider variable scope when dealing with functions, classes, or other PHP constructs in your included files.
Also, it’s worth noting that while this example uses include_once, PHP also offers a similar construct called require_once with minor differences – primarily, require_once will cause a fatal error if the file can’t be found, while include_once only issues a warning but allows the script to continue.
The include construct demonstrates the powerful flexibility PHP provides, enabling you to create cleaner, more modular code. By understanding and correctly using include, you can greatly enhance the readability, reusability, and overall structure of your PHP projects.
Understanding File Inclusion within Functions in PHP
In PHP, the concept of file inclusion plays a crucial role in structuring and organizing code. Specifically, when files are included within a function, it impacts the scope of variables and elements within these files. This section aims to delve deeper into this concept, using an illustrative example and providing additional insights for effective PHP coding.
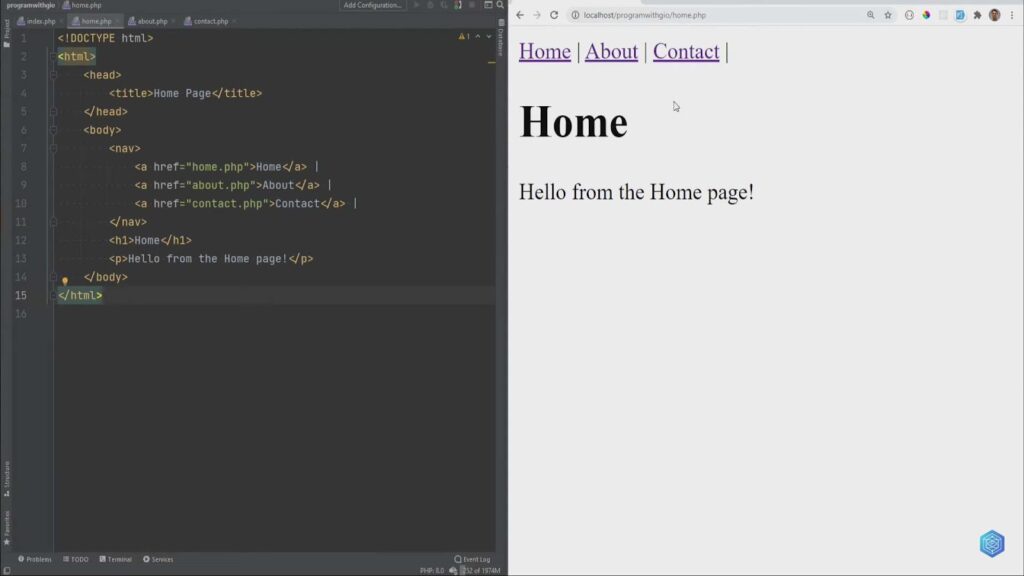
Example of File Inclusion in a Function
Consider a scenario where one needs to include a file within a function in PHP. For instance, imagine a PHP script that includes headers, footers, and a function for rendering articles. The code structure might resemble the following:
Header Inclusion: At the beginning of the script, ‘header.php’ is included.
<?php include 'inc/header.php'; ?>
Function File Inclusion: The script includes ‘functions.php’ once globally.
<?php include_once 'functions.php'; ?>
Article Rendering Function: A function named render_article() is defined, which includes ‘functions.php’.
<?php
function render_article() {
include 'functions.php';
// ... function logic
}
echo render_article();
?>
Footer Inclusion: Finally, ‘footer.php’ is included at the end.
<?php include 'inc/footer.php'; ?>
Conclusion
To conclude, this tutorial has provided you with the essential insights and skills required to seamlessly incorporate code from an external file using the versatile PHP include construct.