The array_filter function in PHP stands out as a straightforward and potent tool for excluding specific elements from an array. By employing a callback function, it discerns which elements should be kept and which should be discarded. This guide delves deeply into utilizing this essential function to optimize and enhance the clarity of your coding practices.
After exploring the intricacies of PHP’s array_filter() function, you may also find it useful to delve into another essential aspect of PHP: substring manipulation. This topic is crucial for effective string handling in various PHP applications.
Efficient Array Filtering Techniques in PHP
Filtering array elements is a common task in PHP programming. Traditionally, this involves manually iterating over each element and determining whether it should be included in the final array.
Consider this example: we have an array $numbers containing [1, 2, 3, 4, 5]. To extract only the odd numbers, a typical approach is to use a foreach loop:
<?php
$numbers = [1, 2, 3, 4, 5];
$odd_numbers = [];
foreach ($numbers as $number) {
if ($number % 2 === 1) {
$odd_numbers[] = $number;
}
}
print_r($odd_numbers);
This code outputs:
Array
(
[0] => 1
[1] => 3
[2] => 5
)
In PHP, handling comparable tasks becomes more streamlined and sophisticated with the array_filter() function. This formidable tool is designed to ease the process of filtering elements in an array, employing a tailored callback function. This approach notably reduces the intricacy of the code, offering an efficient and polished method for array manipulation.
For example, using array_filter():
<?php
$numbers = [1, 2, 3, 4, 5];
$odd_numbers = array_filter(
$numbers,
function ($number) {
return $number % 2 === 1;
}
);
print_r($odd_numbers);
With PHP 7.4 and later, you can simplify this further using the arrow function syntax:
<?php
$numbers = [1, 2, 3, 4, 5];
$odd_numbers = array_filter(
$numbers,
fn ($number) => $number % 2 === 1
);
print_r($odd_numbers);
The array_filter() function takes three parameters: the array to filter ($array), a callback function ($callback), and an optional mode parameter ($mode). It iterates over each element in $array, applying $callback to each element. If the callback returns true, the element is included in the resultant array. The syntax is as follows:
array_filter (
array $array ,
callable|null $callback = null ,
int $mode = 0
) : array
By utilizing array_filter(), developers can write more efficient, readable, and maintainable code for array filtering tasks in PHP.
Applications of PHP’s array_filter() Function
The array_filter() function in PHP is a versatile tool for array manipulation. Let’s explore its various applications through practical examples.
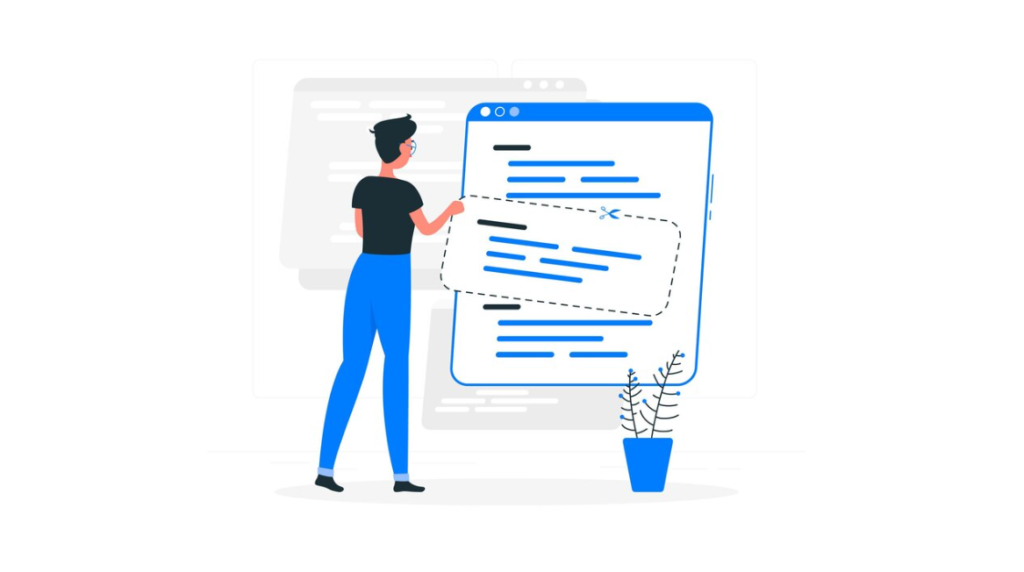
Filtering Even Numbers from an Array
You can use array_filter() to extract even numbers from an array $numbers. The function applies a callback that returns true for even numbers:
<?php
$numbers = [1, 2, 3, 4, 5];
$even_numbers = array_filter(
$numbers,
function ($number) {
return $number % 2 === 0;
}
);
print_r($even_numbers);
Utilizing Class Methods as Callbacks
array_filter() can also employ methods from a class as callbacks. For instance, to filter odd numbers using a class method:
array_filter($items,[$instance,'callback']);
If you have a class with a static method, it can similarly be passed as a callback:
class Odd
{
public function isOdd($num)
{
return $num % 2 === 1;
}
}
The Odd class includes an isOdd() method, which will return a true value when the input is an odd number, and false otherwise. To employ the isOdd() method as a callback for the array_filter() function, utilize the following format:
<?php
class Odd
{
public function isOdd($num)
{
return $num % 2 === 1;
}
}
$numbers = [1, 2, 3, 4, 5];
$odd_numbers = array_filter(
$numbers,
[new Odd(), 'isOdd']
);
print_r($odd_numbers);
When you have a class with a static method, you can provide the static method as the callback function for the array_filter() function like this:
array_filter($array, ['Class','callback']);
As an illustration:
<?php
class Even
{
public static function isEven($num)
{
return $num % 2 === 0;
}
}
The Even class features a static method called isEven(), which returns true if the provided argument is an even number, and false otherwise. The following code snippet demonstrates the use of the isEven() static method as a callback with the array_filter() function in PHP:
$even_numbers = array_filter($numbers, ['Even','isEven']);
Starting from PHP 5.3, if a class implements the __invoke() magic method, it can be utilized as a callable function. For instance, consider the Positive class with the __invoke() method, which returns true for positive numbers and false otherwise:
<?php
class Positive
{
public function __invoke($number)
{
return $number > 0;
}
}
You can then employ an instance of the Positive class as a callback with the array_filter() function to filter out only positive numbers from an array:
<?php
class Positive
{
public function __invoke($number)
{
return $number > 0;
}
}
$numbers = [-1, -2, 0, 1, 2, 3];
$positives = array_filter($numbers, new Positive());
print_r($positives);
Output:
Array
(
[3] => 1
[4] => 2
[5] => 3
)
PHP’s array_filter() Function for Advanced Element Filtering
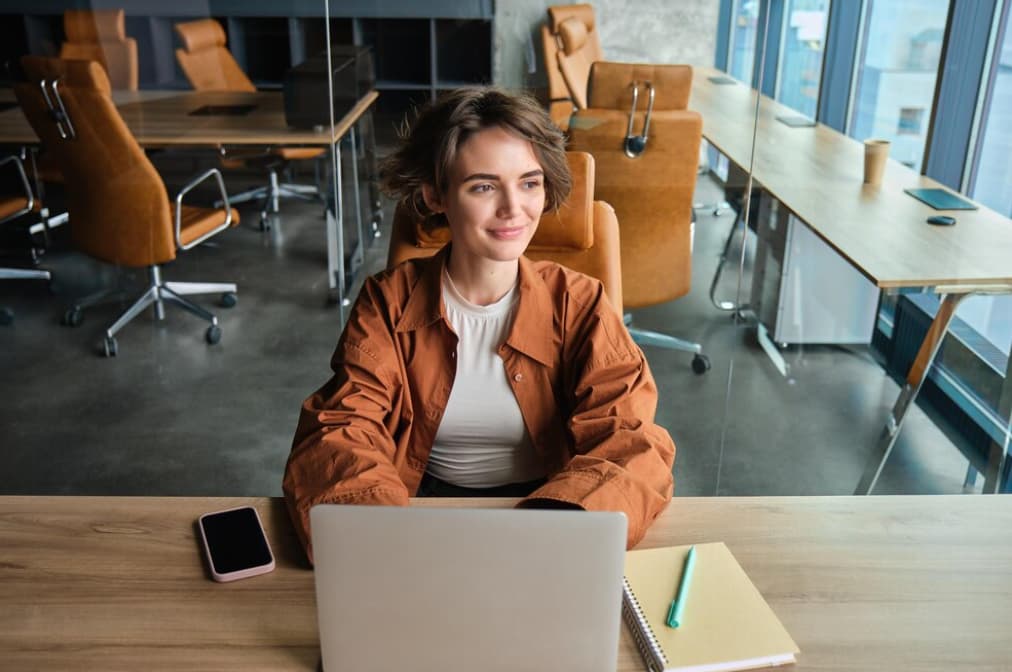
When working with arrays in PHP, a common requirement is to filter their elements based on specific criteria. The array_filter() function is a versatile tool for this purpose, allowing you to apply a callback function to each element for filtering. However, there are scenarios where you might need more than just the element’s value for effective filtering.
Consider a scenario where the keys of the array elements are crucial for your filtering logic. PHP’s array_filter() function caters to this need by enabling you to pass the key instead of the value to your callback function. This is achieved by using ARRAY_FILTER_USE_KEY as the third argument in the array_filter() function.
Here’s an illustrative example:
<?php
$inputs = [
'first' => 'John',
'last' => 'Doe',
'password' => 'secret',
'email' => '[email protected]'
];
$filtered = array_filter(
$inputs,
fn ($key) => $key !== 'password',
ARRAY_FILTER_USE_KEY
);
print_r($filtered);
This snippet filters out the element with the key ‘password’, resulting in the following output:
Array
(
[first] => John
[last] => Doe
[email] => [email protected]
)
But what if your filtering logic requires both the key and the value of the array elements? PHP accommodates this scenario too. By passing ARRAY_FILTER_USE_BOTH as the third argument to array_filter(), you can access both the key and value in your callback function. Here’s an example:
<?php
$inputs = [
'first' => 'John',
'last' => 'Doe',
'password' => 'secret',
'email' => ''
];
$filtered = array_filter(
$inputs,
fn ($value, $key) => $value !== '' && $key !== 'password',
ARRAY_FILTER_USE_BOTH
);
print_r($filtered);
The output for this code will be:
Array
(
[first] => John
[last] => Doe
)
Conclusion
This tutorial demonstrates the flexibility of PHP’s array_filter() function in filtering array elements. By leveraging the capability to pass either the key, value, or both to the callback function, you can implement complex filtering logic tailored to your specific needs.